Programmatic example with MARS/GRIB input#
[1]:
%%javascript
// leave this in to disable autoscroll in Jupyter notebook
IPython.OutputArea.prototype._should_scroll = function(lines) {
return false;
}
Imports#
[2]:
import os
from pathlib import Path
import json
import numpy as np
import fcpy
Load data#
[3]:
if (Path().home() / ".ecmwfapirc").exists():
fpath = "../samples/cases/hplp_ml_q.json"
request = json.load(open(fpath))["mars"]
ds = fcpy.fetch_mars_dataset(request)
else:
ds = fcpy.open_dataset("../data/mars.grib")
ds = ds.isel(hybrid=[0, 1])
ds
In , overriding the default value (cache=True) with cache=False is not recommended.
[3]:
<xarray.Dataset> Dimensions: (time: 1, step: 1, hybrid: 2, values: 654400) Coordinates: * time (time) datetime64[ns] 2020-07-21 * step (step) timedelta64[ns] 00:00:00 * hybrid (hybrid) float64 1.0 2.0 latitude (values) float64 ... longitude (values) float64 ... valid_time (time, step) datetime64[ns] ... Dimensions without coordinates: values Data variables: q (time, step, hybrid, values) float64 ... Attributes: GRIB_edition: 2 GRIB_centre: ecmf GRIB_centreDescription: European Centre for Medium-Range Weather Forecasts GRIB_subCentre: 0 Conventions: CF-1.7 institution: European Centre for Medium-Range Weather Forecasts history: 2023-05-08T18:01 GRIB to CDM+CF via cfgrib-0.9.1... path: ../data/mars.grib
Define and run experiment#
[4]:
suite = fcpy.Suite(
ds,
baseline=fcpy.Float(bits=32),
compressors=[fcpy.LinQuantization(), fcpy.Round()],
metrics=[fcpy.Difference, fcpy.AbsoluteError],
bits=[13, 14, 15, 16, 17, 18, 19, 20, 21, 22],
max_chunk_size_bytes=451 * 900 * 6,
skip_histograms=True,
)
Activating project at `~/work/field-compression/field-compression`
WARNING: method definition for == at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:68 declares type variable T but does not use it.
WARNING: method definition for getindex at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:120 declares type variable T but does not use it.
WARNING: method definition for getindex at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:120 declares type variable P but does not use it.
WARNING: method definition for canonicalize at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:240 declares type variable L but does not use it.
WARNING: method definition for canonicalize at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:241 declares type variable L but does not use it.
Lineplot: evaluate the effect of bits#
[5]:
suite.lineplot(fcpy.AbsoluteError, reduction="max")
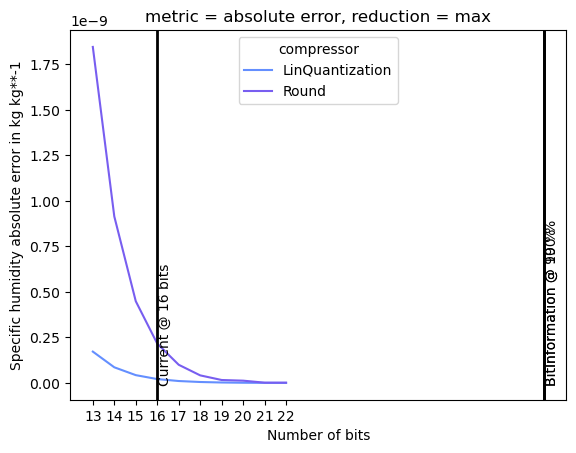
Boxplot: evaluate statistical characteristics of errors#
[6]:
bits = 13
suite.boxplot(metric=fcpy.Difference, compressor="LinQuantization", bits=bits)
suite.boxplot(metric=fcpy.Difference, compressor="Round", bits=bits)
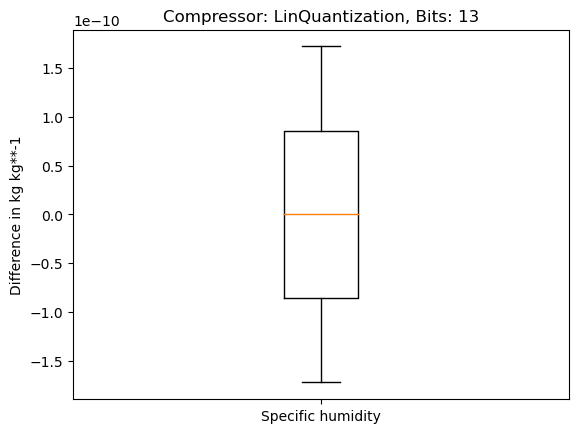
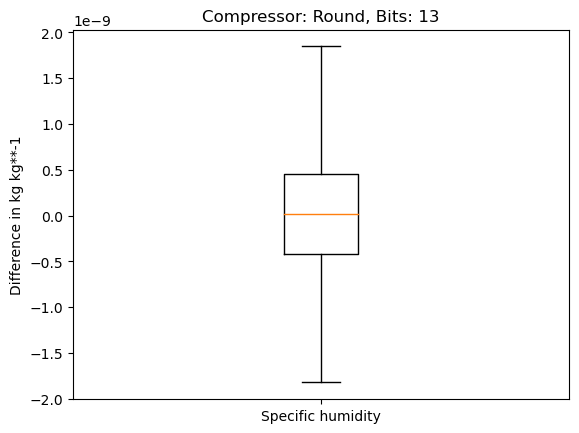
Spatial plots: evaluate the spatial distribution of errors#
[7]:
if not os.getenv("SPHINX_BUILD"):
# Here we compare the spatial change of specific humidity
# by rounding values to 8 bits
fcpy.spatialplot(
ds=ds,
baseline=fcpy.Float(bits=32),
var_name="q",
compressor=fcpy.Round(bits=6),
metric=fcpy.Difference,
latitude=0,
longitude=0,
hybrid=1,
step=np.timedelta64(0, "ns"),
third_dim="hybrid",
)
[ ]: