Programmatic example with NetCDF input#
[1]:
%%javascript
// leave this in to disable autoscroll in Jupyter notebook
IPython.OutputArea.prototype._should_scroll = function(lines) {
return false;
}
Imports#
[2]:
import fcpy
Load data#
[3]:
# the `load_dataset' option is currently unavalable due to data handling system move.
# ds = fcpy.load_dataset(model='atmospheric-model', var_names=['2t', 'q', 'sst'], levels=list(range(1, 10)))
# The `data` folder contains two sample NetCDF files.
# Here we load specific humidity from CAMS at 32 bits
fpath = "../data/*_q_*.nc"
ds = fcpy.open_dataset(fpath)
ds = ds[["q"]] # Select only q as this dataset contains more vars...
ds
[3]:
<xarray.Dataset> Dimensions: (time: 1, lev: 137, lat: 451, lon: 900) Coordinates: * time (time) datetime64[ns] 2019-12-01T12:00:00 * lon (lon) float64 0.0 0.4 0.8 1.2 1.6 ... 358.0 358.4 358.8 359.2 359.6 * lat (lat) float64 -90.0 -89.6 -89.2 -88.8 -88.4 ... 88.8 89.2 89.6 90.0 * lev (lev) float64 1.0 2.0 3.0 4.0 5.0 ... 133.0 134.0 135.0 136.0 137.0 Data variables: q (time, lev, lat, lon) float32 dask.array<chunksize=(1, 137, 451, 900), meta=np.ndarray> Attributes: CDI: Climate Data Interface version 1.9.8 (https://mpimet.mpg.de... Conventions: CF-1.6 history: Mon Feb 15 18:37:28 2021: cdo -f nc4 copy tmp.grib data/mil... institution: European Centre for Medium-Range Weather Forecasts CDO: Climate Data Operators version 1.9.8 (https://mpimet.mpg.de...
Define and run experiment#
[4]:
suite = fcpy.Suite(
ds,
baseline=fcpy.Float(bits=32),
compressors=[fcpy.LinQuantization(), fcpy.Round()],
metrics=[fcpy.Difference, fcpy.AbsoluteError],
bits=[13],
max_chunk_size_bytes=451 * 900 * 6,
skip_histograms=False,
)
/home/runner/work/field-compression/field-compression/fcpy/suite.py:155: UserWarning: q: chunk dims adjusted to ['lat', 'lon']
warnings.warn(f"{da.name}: chunk dims adjusted to {max_chunk_dims}")
0%| | 0/137 [00:00<?, ?it/s] Activating project at `~/work/field-compression/field-compression`
WARNING: method definition for == at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:68 declares type variable T but does not use it.
WARNING: method definition for getindex at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:120 declares type variable T but does not use it.
WARNING: method definition for getindex at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:120 declares type variable P but does not use it.
WARNING: method definition for canonicalize at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:240 declares type variable L but does not use it.
WARNING: method definition for canonicalize at /usr/share/miniconda/envs/fcpy/share/julia/packages/ChainRulesCore/ctmSK/src/tangent_types/tangent.jl:241 declares type variable L but does not use it.
1%|▏ | 2/137 [00:52<48:54, 21.73s/it]
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0522301181481453e-06, 4.548326614894904e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0522301181481453e-06, 4.548326614894904e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0522301181481453e-06, 4.548326614894904e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0523945093154907e-06, 4.548579454421997e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.125241055888182e-06, 4.5921033233753406e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.125241055888182e-06, 4.5921033233753406e-06])
2%|▏ | 3/137 [00:52<26:32, 11.89s/it]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.125241055888182e-06, 4.5921033233753406e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.125037670135498e-06, 4.59328293800354e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2289827964195865e-06, 4.619874744093977e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2289827964195865e-06, 4.619874744093977e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2289827964195865e-06, 4.619874744093977e-06])
4%|▎ | 5/137 [00:53<10:20, 4.70s/it]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.2293457984924316e-06, 4.6193599700927734e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.3230717286205618e-06, 4.66631217932445e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.3230717286205618e-06, 4.66631217932445e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.3230717286205618e-06, 4.66631217932445e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.323409378528595e-06, 4.667788743972778e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.5890789200057043e-06, 4.747750608657952e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.5890789200057043e-06, 4.747750608657952e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.5890789200057043e-06, 4.747750608657952e-06])
5%|▌ | 7/137 [00:53<04:42, 2.18s/it]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.5888363122940063e-06, 4.7460198402404785e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.694198886070808e-06, 4.773798536916729e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.694198886070808e-06, 4.773798536916729e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.694198886070808e-06, 4.773798536916729e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.6940757632255554e-06, 4.772096872329712e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.72851514435024e-06, 4.792062100023031e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.72851514435024e-06, 4.792062100023031e-06])
6%|▌ | 8/137 [00:53<03:17, 1.53s/it]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.72851514435024e-06, 4.792062100023031e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.7285346984863281e-06, 4.7907233238220215e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.8230480236525182e-06, 4.790477760252543e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.8230480236525182e-06, 4.790477760252543e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.8230480236525182e-06, 4.790477760252543e-06])
7%|▋ | 10/137 [00:53<01:42, 1.23it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.8225982785224915e-06, 4.7907233238220215e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.100172878272133e-06, 4.7554522097925656e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.100172878272133e-06, 4.7554522097925656e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.100172878272133e-06, 4.7554522097925656e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.100132405757904e-06, 4.755333065986633e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.431908342259703e-06, 4.717675892607076e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.431908342259703e-06, 4.717675892607076e-06])
8%|▊ | 11/137 [00:54<01:16, 1.64it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.431908342259703e-06, 4.717675892607076e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.4316832423210144e-06, 4.718080163002014e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.7860419322678354e-06, 4.687765795097221e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.7860419322678354e-06, 4.687765795097221e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.7860419322678354e-06, 4.687765795097221e-06])
9%|▉ | 13/137 [00:54<00:46, 2.65it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.786051481962204e-06, 4.687346518039703e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.1883046176517382e-06, 4.651170911529334e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.1883046176517382e-06, 4.651170911529334e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.1883046176517382e-06, 4.651170911529334e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.1883828341960907e-06, 4.6510249376297e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.4696747661655536e-06, 4.608740255207522e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.4696747661655536e-06, 4.608740255207522e-06])
10%|█ | 14/137 [00:54<00:38, 3.22it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.4696747661655536e-06, 4.608740255207522e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.469642251729965e-06, 4.609115421772003e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.7490763133973815e-06, 4.5811448217136785e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.7490763133973815e-06, 4.5811448217136785e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.7490763133973815e-06, 4.5811448217136785e-06])
12%|█▏ | 16/137 [00:54<00:27, 4.32it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.7490390241146088e-06, 4.581175744533539e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.995706720161252e-06, 4.533689207164571e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.995706720161252e-06, 4.533689207164571e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.995706720161252e-06, 4.533689207164571e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.9958395063877106e-06, 4.533678293228149e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.861576260533184e-06, 4.4628559408010915e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.861576260533184e-06, 4.4628559408010915e-06])
12%|█▏ | 17/137 [00:55<00:25, 4.78it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.861576260533184e-06, 4.4628559408010915e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.861729055643082e-06, 4.462897777557373e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.7494210118893534e-06, 4.467015969567001e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.7494210118893534e-06, 4.467015969567001e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.7494210118893534e-06, 4.467015969567001e-06])
14%|█▍ | 19/137 [00:55<00:21, 5.48it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.7495046854019165e-06, 4.466623067855835e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.6435562833503354e-06, 4.463335699256277e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.6435562833503354e-06, 4.463335699256277e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.6435562833503354e-06, 4.463335699256277e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.643333911895752e-06, 4.462897777557373e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.513923957143561e-06, 4.461072876438266e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.513923957143561e-06, 4.461072876438266e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.513923957143561e-06, 4.461072876438266e-06])
15%|█▌ | 21/137 [00:55<00:19, 6.02it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.5138800740242004e-06, 4.461035132408142e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.4046290693368064e-06, 4.466321570362197e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.4046290693368064e-06, 4.466321570362197e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.4046290693368064e-06, 4.466321570362197e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.4044496715068817e-06, 4.466623067855835e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.305642849227297e-06, 4.4940893531020265e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.305642849227297e-06, 4.4940893531020265e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.305642849227297e-06, 4.4940893531020265e-06])
17%|█▋ | 23/137 [00:56<00:18, 6.14it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.305729478597641e-06, 4.493631422519684e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.222206032660324e-06, 4.527914825303014e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.222206032660324e-06, 4.527914825303014e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.222206032660324e-06, 4.527914825303014e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.2223761081695557e-06, 4.5280903577804565e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.156796083203517e-06, 4.56218549516052e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.156796083203517e-06, 4.56218549516052e-06])
18%|█▊ | 24/137 [00:56<00:18, 6.17it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.156796083203517e-06, 4.56218549516052e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.1567178666591644e-06, 4.562549293041229e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.1039946861710632e-06, 4.578624611895066e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.1039946861710632e-06, 4.578624611895066e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.1039946861710632e-06, 4.578624611895066e-06])
19%|█▉ | 26/137 [00:56<00:17, 6.27it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.10409814119339e-06, 4.578381776809692e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.059771643165732e-06, 4.591241577145411e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.059771643165732e-06, 4.591241577145411e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.059771643165732e-06, 4.591241577145411e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.0598603188991547e-06, 4.591420292854309e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.0062046789680608e-06, 4.593578978528967e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.0062046789680608e-06, 4.593578978528967e-06])
20%|█▉ | 27/137 [00:56<00:17, 6.30it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.0062046789680608e-06, 4.593578978528967e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.0063092708587646e-06, 4.59328293800354e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.9526790967793204e-06, 4.589483523886884e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.9526790967793204e-06, 4.589483523886884e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.9526790967793204e-06, 4.589483523886884e-06])
21%|██ | 29/137 [00:56<00:17, 6.12it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.9527582228183746e-06, 4.589557647705078e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.9266834644658957e-06, 4.5786414375470486e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.9266834644658957e-06, 4.5786414375470486e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.9266834644658957e-06, 4.5786414375470486e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.9266811907291412e-06, 4.578381776809692e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.8666552225331543e-06, 4.546167929220246e-06])
22%|██▏ | 30/137 [00:57<00:17, 6.16it/s]
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.8666552225331543e-06, 4.546167929220246e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.8666552225331543e-06, 4.546167929220246e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.866610884666443e-06, 4.545785486698151e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.8133756586612435e-06, 4.505715878622141e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.8133756586612435e-06, 4.505715878622141e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.8133756586612435e-06, 4.505715878622141e-06])
23%|██▎ | 32/137 [00:57<00:16, 6.29it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.8135254979133606e-06, 4.505738615989685e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.7805510853795568e-06, 4.454643203644082e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.7805510853795568e-06, 4.454643203644082e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.7805510853795568e-06, 4.454643203644082e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.780463546514511e-06, 4.454515874385834e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.769791763057583e-06, 4.3824170461448375e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.769791763057583e-06, 4.3824170461448375e-06])
24%|██▍ | 33/137 [00:57<00:16, 6.23it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.769791763057583e-06, 4.3824170461448375e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.769753336906433e-06, 4.382804036140442e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.769852244455251e-06, 4.304451067582704e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.769852244455251e-06, 4.304451067582704e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.769852244455251e-06, 4.304451067582704e-06])
26%|██▌ | 35/137 [00:57<00:15, 6.46it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.769753336906433e-06, 4.304572939872742e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.771099389065057e-06, 4.237938355799997e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.771099389065057e-06, 4.237938355799997e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.771099389065057e-06, 4.237938355799997e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.7711503207683563e-06, 4.237517714500427e-06])
36%|███▋ | 50/137 [01:00<00:13, 6.25it/s]
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2855675777245779e-06, 4.57707255918649e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2855675777245779e-06, 4.57707255918649e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2855675777245779e-06, 4.57707255918649e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.2852251529693604e-06, 4.578381776809692e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.158373493126419e-06, 5.264952051220462e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.158373493126419e-06, 5.264952051220462e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.158373493126419e-06, 5.264952051220462e-06])
38%|███▊ | 52/137 [01:00<00:13, 6.17it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.1585652828216553e-06, 5.2638351917266846e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0959125802401104e-06, 5.882356163056102e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0959125802401104e-06, 5.882356163056102e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0959125802401104e-06, 5.882356163056102e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0961666703224182e-06, 5.882233381271362e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0540567245698185e-06, 6.055937319615623e-06])
39%|███▊ | 53/137 [01:00<00:13, 6.18it/s]
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0540567245698185e-06, 6.055937319615623e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0540567245698185e-06, 6.055937319615623e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0542571544647217e-06, 6.057322025299072e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0464611932547996e-06, 5.491593583428767e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0464611932547996e-06, 5.491593583428767e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0464611932547996e-06, 5.491593583428767e-06])
40%|████ | 55/137 [01:01<00:13, 6.19it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0468065738677979e-06, 5.491077899932861e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.209982418818981e-07, 7.076708698150469e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.209982418818981e-07, 7.076708698150469e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.209982418818981e-07, 7.076708698150469e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [5.210749804973602e-07, 7.078051567077637e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.3294529682971188e-07, 4.402432750794105e-06])
41%|████ | 56/137 [01:01<00:13, 6.19it/s]
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.3294529682971188e-07, 4.402432750794105e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.3294529682971188e-07, 4.402432750794105e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.3294705897569656e-07, 4.403293132781982e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.4620158001198433e-07, 4.343768068792997e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.4620158001198433e-07, 4.343768068792997e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.4620158001198433e-07, 4.343768068792997e-06])
42%|████▏ | 58/137 [01:01<00:12, 6.18it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.461019903421402e-07, 4.343688488006592e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.412820603898581e-07, 4.700554654846201e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.412820603898581e-07, 4.700554654846201e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.412820603898581e-07, 4.700554654846201e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.413297235965729e-07, 4.7013163566589355e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.171544898985303e-07, 4.443074885784881e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.171544898985303e-07, 4.443074885784881e-06])
43%|████▎ | 59/137 [01:01<00:12, 6.22it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.171544898985303e-07, 4.443074885784881e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [5.173496901988983e-07, 4.4442713260650635e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.751008641003864e-07, 4.683110546466196e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.751008641003864e-07, 4.683110546466196e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.751008641003864e-07, 4.683110091718845e-06])
45%|████▍ | 61/137 [01:02<00:12, 6.22it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [6.752088665962219e-07, 4.682689905166626e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.377642757295689e-07, 4.9948225750995334e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.377642757295689e-07, 4.9948225750995334e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.377642757295689e-07, 4.9948225750995334e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [8.377246558666229e-07, 4.995614290237427e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.528415378350473e-07, 6.5232084125455e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.528415378350473e-07, 6.5232084125455e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.528415378350473e-07, 6.5232084125455e-06])
46%|████▌ | 63/137 [01:02<00:14, 5.21it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [8.526258170604706e-07, 6.5229833126068115e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.593338639504509e-07, 9.071186468645465e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.593338639504509e-07, 9.071186468645465e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.593338639504509e-07, 9.071186468645465e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [6.593763828277588e-07, 9.074807167053223e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.822983884077985e-07, 8.731875823286828e-06])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.822983884077985e-07, 8.731875823286828e-06])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.822983884077985e-07, 8.731874913792126e-06])
47%|████▋ | 65/137 [01:02<00:12, 5.85it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [9.825453162193298e-07, 8.732080459594727e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0079162393594743e-06, 1.1395792171242647e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0079162393594743e-06, 1.1395792171242647e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0079162393594743e-06, 1.1395792171242647e-05])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0076910257339478e-06, 1.1399388313293457e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0591282944005798e-06, 1.5105805687198881e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0591282944005798e-06, 1.5105805687198881e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0591282944005798e-06, 1.5105805687198881e-05])
49%|████▉ | 67/137 [01:03<00:11, 6.22it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.058913767337799e-06, 1.51023268699646e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1345157417963492e-06, 1.8826494851964526e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1345157417963492e-06, 1.8826494851964526e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1345157417963492e-06, 1.8826494851964526e-05])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.1343508958816528e-06, 1.882016658782959e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2500016737249098e-06, 2.538259286666289e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2500016737249098e-06, 2.538259286666289e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2500016737249098e-06, 2.538259286666289e-05])
50%|█████ | 69/137 [01:03<00:10, 6.37it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.2498348951339722e-06, 2.537667751312256e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.3425875522443675e-06, 3.5722307075047866e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.3425875522443675e-06, 3.5722307075047866e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.3425875522443675e-06, 3.5722307075047866e-05])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.341104507446289e-06, 3.5762786865234375e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.819051456361194e-07, 5.1220755267422646e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.819051456361194e-07, 5.1220755267422646e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.819051456361194e-07, 5.1220755267422646e-05])
52%|█████▏ | 71/137 [01:03<00:10, 6.46it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [9.834766387939453e-07, 5.125999450683594e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.616374434997851e-07, 7.31356703909114e-05])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.616374434997851e-07, 7.31356703909114e-05])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.616374434997851e-07, 7.31356703909114e-05])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [6.612390279769897e-07, 7.319450378417969e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.6326861757297593e-07, 0.000109518674435094])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.6326861757297593e-07, 0.000109518674435094])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.6326861757297593e-07, 0.000109518674435094])
53%|█████▎ | 73/137 [01:04<00:09, 6.51it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.635642886161804e-07, 0.00010943412780761719])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.268921892005892e-07, 0.00014867489517200738])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.268921892005892e-07, 0.00014867489517200738])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.268921892005892e-07, 0.00014867489517200738])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.2665987014770508e-07, 0.000148773193359375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.1033338271081448e-07, 2.009328454732895e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.1033338271081448e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.8631121179168986e-07, 0.0002007141592912376])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.8631121179168986e-07, 0.0002007141592912376])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.8631121179168986e-07, 0.0002007141592912376])
55%|█████▍ | 75/137 [01:04<00:09, 6.59it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.862645149230957e-07, 0.00020074844360351562])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.383749233558774e-07, 2.3063330445438623e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.383749233558774e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.2680756589797966e-07, 0.00026751996483653784])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.2680756589797966e-07, 0.00026751996483653784])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.2680756589797966e-07, 0.00026751996483653784])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.2689422369003296e-07, 0.00026798248291015625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-4.62518073618412e-07, 2.3840402718633413e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 4.62518073618412e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.022464400397439e-07, 0.00035638813278637826])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.022464400397439e-07, 0.00035638813278637826])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.022464400397439e-07, 0.00035638813278637826])
56%|█████▌ | 77/137 [01:04<00:10, 5.49it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.0209699869155884e-07, 0.0003566741943359375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-4.4296029955148697e-07, 4.61208401247859e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 4.61208401247859e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000226424722314e-08, 0.0004635036748368293])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000226424722314e-08, 0.0004635036748368293])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000226424722314e-08, 0.0004635036748368293])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0011717677116394e-08, 0.0004634857177734375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-4.734611138701439e-07, 4.7471257857978344e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 4.7471257857978344e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000026584577881e-08, 0.0005854322807863355])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000026584577881e-08, 0.0005854322807863355])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000026584577881e-08, 0.0005854322807863355])
58%|█████▊ | 79/137 [01:05<00:09, 5.93it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0011717677116394e-08, 0.0005855560302734375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-4.833564162254333e-07, 7.897615432739258e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.897615432739258e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000099415208297e-08, 0.0007311435183510184])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000099415208297e-08, 0.0007311435183510184])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0000099415208297e-08, 0.0007311435183510184])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0011717677116394e-08, 0.0007305145263671875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-9.017530828714371e-07, 9.50181856751442e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 9.50181856751442e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1563454194174483e-08, 0.000907466805074364])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1563454194174483e-08, 0.000907466805074364])
58%|█████▊ | 80/137 [01:05<00:09, 6.08it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1563454194174483e-08, 0.000907466805074364])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.155422069132328e-08, 0.00090789794921875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-9.459326975047588e-07, 9.504146873950958e-07])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 9.504146873950958e-07])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.451412945385755e-08, 0.0011311575071886182])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.451412945385755e-08, 0.0011311575071886182])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.451412945385755e-08, 0.0011311575071886182])
60%|█████▉ | 82/137 [01:05<00:09, 6.03it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.4522811397910118e-08, 0.001132965087890625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.8075807020068169e-06, 1.7517013475298882e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.8075807020068169e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0063745392585588e-08, 0.0013909898698329926])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0063745392585588e-08, 0.0013909898698329926])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0063745392585588e-08, 0.0013909898698329926])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0069925338029861e-08, 0.001392364501953125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.6208505257964134e-06, 1.5044352039694786e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.6208505257964134e-06])
61%|██████ | 83/137 [01:05<00:08, 6.05it/s]
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2422457729144298e-08, 0.001663318951614201])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2422457729144298e-08, 0.001663318951614201])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2422457729144298e-08, 0.001663318951614201])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.2427335605025291e-08, 0.0016632080078125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.8524006009101868e-06, 1.857173629105091e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.857173629105091e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1178180159276963e-08, 0.001965682953596115])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1178180159276963e-08, 0.001965682953596115])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1178180159276963e-08, 0.001965682953596115])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.1999509297311306e-07, 1.200241968035698e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.200241968035698e-07])
62%|██████▏ | 85/137 [01:06<00:08, 6.09it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.1175870895385742e-08, 0.0019683837890625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.7008354663848877e-06, 1.9069993868470192e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.7008354663848877e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.976340216624521e-08, 0.0023388906847685575])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.976340216624521e-08, 0.0023388906847685575])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.976340216624521e-08, 0.0023388906847685575])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.4278339222073555e-07, 1.4281249605119228e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.4281249605119228e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [6.984919309616089e-08, 0.00234222412109375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.3334363251924515e-06, 3.0228402465581894e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.3334363251924515e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.261036051251722e-08, 0.0027658389881253242])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.261036051251722e-08, 0.0027658389881253242])
63%|██████▎ | 86/137 [01:06<00:08, 6.16it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.261036051251722e-08, 0.0027658389881253242])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.6883132047951221e-07, 1.6886042430996895e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.6886042430996895e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [5.2619725465774536e-08, 0.00276947021484375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.7010759115219116e-06, 3.7795398384332657e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.7795398384332657e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.5273164422069385e-07, 0.003196049015969038])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.5273164422069385e-07, 0.003196049015969038])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.5273164422069385e-07, 0.003196049015969038])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.9511207938194275e-07, 1.9511207938194275e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.9511207938194275e-07])
64%|██████▍ | 88/137 [01:06<00:07, 6.23it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.5273690223693848e-07, 0.00319671630859375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.797002136707306e-06, 3.5043340176343918e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.797002136707306e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.602663980473153e-07, 0.003613774897530675])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.602663980473153e-07, 0.003613774897530675])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.602663980473153e-07, 0.003613774897530675])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-2.207234501838684e-07, 2.2060703486204147e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 2.207234501838684e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.604218363761902e-07, 0.0036163330078125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.814697265625e-06, 3.804219886660576e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.814697265625e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.815589030229603e-07, 0.004038688726723194])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.815589030229603e-07, 0.004038688726723194])
65%|██████▍ | 89/137 [01:06<00:07, 6.27it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.815589030229603e-07, 0.004038688726723194])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-2.4656765162944794e-07, 2.4656765162944794e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 2.4656765162944794e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.816079020500183e-07, 0.0040435791015625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-4.890374839305878e-06, 3.813067451119423e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 4.890374839305878e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.4899944744684035e-07, 0.004459286108613014])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.4899944744684035e-07, 0.004459286108613014])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.4899944744684035e-07, 0.004459286108613014])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-2.7220085030421615e-07, 2.7223723009228706e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 2.7223723009228706e-07])
66%|██████▋ | 91/137 [01:07<00:07, 6.23it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.4901161193847656e-07, 0.00445556640625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-6.983987987041473e-06, 3.814464434981346e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 6.983987987041473e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.4247142132717272e-07, 0.0049603162333369255])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.4247142132717272e-07, 0.0049603162333369255])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.4247142132717272e-07, 0.0049603162333369255])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-3.029126673936844e-07, 3.028544597327709e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 3.029126673936844e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.424923539161682e-07, 0.0049591064453125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.613096386194229e-06, 6.977934390306473e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.613096386194229e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.876344744137896e-07, 0.00556230777874589])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.876344744137896e-07, 0.00556230777874589])
67%|██████▋ | 92/137 [01:07<00:07, 6.38it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [5.876344744137896e-07, 0.00556230777874589])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-3.395252861082554e-07, 3.396999090909958e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 3.396999090909958e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [5.867332220077515e-07, 0.0055694580078125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.406808435916901e-06, 7.525086402893066e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.525086402893066e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.7197850183947594e-06, 0.006085578817874193])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.7197850183947594e-06, 0.006085578817874193])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.7197850183947594e-06, 0.006085578817874193])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-3.7159770727157593e-07, 3.71481291949749e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 3.7159770727157593e-07])
69%|██████▊ | 94/137 [01:07<00:06, 6.57it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.7210841178894043e-06, 0.0060882568359375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.624272257089615e-06, 7.622409611940384e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.624272257089615e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.634932555651176e-07, 0.006721201352775097])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.634932555651176e-07, 0.006721201352775097])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.634932555651176e-07, 0.006721201352775097])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-4.102475941181183e-07, 4.103640094399452e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 4.103640094399452e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [8.642673492431641e-07, 0.0067138671875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.623806595802307e-06, 7.61914998292923e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.623806595802307e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.310208841066924e-07, 0.00752916419878602])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.310208841066924e-07, 0.00752916419878602])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.310208841066924e-07, 0.00752916419878602])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-4.5960769057273865e-07, 4.596658982336521e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 4.596658982336521e-07])
70%|███████ | 96/137 [01:07<00:06, 6.66it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [8.307397365570068e-07, 0.0075225830078125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.621943950653076e-06, 7.6284632086753845e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.6284632086753845e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.2151379514289147e-07, 0.00824328139424324])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.2151379514289147e-07, 0.00824328139424324])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.2151379514289147e-07, 0.00824328139424324])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-5.033798515796661e-07, 5.032634362578392e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 5.033798515796661e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.213062882423401e-07, 0.00823974609375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.628928869962692e-06, 7.62939453125e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.62939453125e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.916483114428047e-07, 0.008857328444719315])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.916483114428047e-07, 0.008857328444719315])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.916483114428047e-07, 0.008857328444719315])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-5.406909622251987e-07, 5.407491698861122e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 5.407491698861122e-07])
72%|███████▏ | 98/137 [01:08<00:05, 6.72it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.9185245037078857e-07, 0.00885009765625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-7.628928869962692e-06, 7.62939453125e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 7.62939453125e-06])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0005107853316986e-08, 0.009448678232729435])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0005107853316986e-08, 0.009448678232729435])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0005107853316986e-08, 0.009448678232729435])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-5.769543349742889e-07, 5.769543349742889e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 5.769543349742889e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0011717677116394e-08, 0.00946044921875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.2313015758991241e-05, 1.0418705642223358e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.2313015758991241e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.17300347213245e-08, 0.00984727032482624])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.17300347213245e-08, 0.00984727032482624])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.17300347213245e-08, 0.00984727032482624])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-6.011687219142914e-07, 6.011687219142914e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 6.011687219142914e-07])
73%|███████▎ | 100/137 [01:08<00:05, 6.77it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.1728843674063683e-08, 0.009857177734375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.4774501323699951e-05, 9.136274456977844e-06])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.4774501323699951e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1959319756726927e-08, 0.010651803575456142])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1959319756726927e-08, 0.010651803575456142])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1959319756726927e-08, 0.010651803575456142])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-6.505288183689117e-07, 6.502959877252579e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 6.505288183689117e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.1961674317717552e-08, 0.010650634765625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5153549611568451e-05, 1.4426186680793762e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5153549611568451e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.0136589284902584e-07, 0.011526549234986305])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.0136589284902584e-07, 0.011526549234986305])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.0136589284902584e-07, 0.011526549234986305])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-7.03614205121994e-07, 7.038470357656479e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 7.038470357656479e-07])
74%|███████▍ | 102/137 [01:08<00:05, 6.63it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.0116567611694336e-07, 0.01153564453125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5188939869403839e-05, 1.5245750546455383e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5245750546455383e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.3458229609095724e-07, 0.012178774923086166])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.3458229609095724e-07, 0.012178774923086166])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.3458229609095724e-07, 0.012178774923086166])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-7.436610758304596e-07, 7.436610758304596e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 7.436610758304596e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.343448042869568e-07, 0.012176513671875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5194527804851532e-05, 1.5230849385261536e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5230849385261536e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1077834649597662e-08, 0.012968861497938633])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1077834649597662e-08, 0.012968861497938633])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1077834649597662e-08, 0.012968861497938633])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-7.920898497104645e-07, 7.920898497104645e-07])
75%|███████▌ | 103/137 [01:08<00:05, 6.64it/s]
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 7.920898497104645e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.1088559404015541e-08, 0.012969970703125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5228986740112305e-05, 1.524854451417923e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.524854451417923e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.99999993922529e-09, 0.013690117746591568])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.99999993922529e-09, 0.013690117746591568])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.99999993922529e-09, 0.013690117746591568])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-8.35862010717392e-07, 8.35862010717392e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 8.35862010717392e-07])
77%|███████▋ | 105/137 [01:09<00:04, 6.54it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0011717677116394e-08, 0.013702392578125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.5255063772201538e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0619240597975477e-08, 0.014276280999183655])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0619240597975477e-08, 0.014276280999183655])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0619240597975477e-08, 0.014276280999183655])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-8.717179298400879e-07, 8.717179298400879e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 8.717179298400879e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0622898116707802e-08, 0.0142822265625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5257857739925385e-05, 1.5255995094776154e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5257857739925385e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.6254933399295624e-08, 0.014798803254961967])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.6254933399295624e-08, 0.014798803254961967])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.6254933399295624e-08, 0.014798803254961967])
77%|███████▋ | 106/137 [01:09<00:04, 6.61it/s]
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-9.033828973770142e-07, 9.033828973770142e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 9.033828973770142e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.6239937394857407e-08, 0.014801025390625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5257857739925385e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.704818153314136e-08, 0.015400518663227558])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.704818153314136e-08, 0.015400518663227558])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.704818153314136e-08, 0.015400518663227558])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-9.406358003616333e-07, 9.406358003616333e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 9.406358003616333e-07])
79%|███████▉ | 108/137 [01:09<00:04, 6.71it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.705484464764595e-08, 0.015411376953125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5257857739925385e-05, 1.5257857739925385e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5257857739925385e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0030060337840041e-08, 0.01612737774848938])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0030060337840041e-08, 0.01612737774848938])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.0030060337840041e-08, 0.01612737774848938])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-9.844079613685608e-07, 9.846407920122147e-07])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 9.846407920122147e-07])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.0040821507573128e-08, 0.01611328125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.6303732991218567e-05, 1.5257857739925385e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.6303732991218567e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1238472374941466e-08, 0.016749197617173195])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1238472374941466e-08, 0.016749197617173195])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.1238472374941466e-08, 0.016749197617173195])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.0225921869277954e-06, 1.0225921869277954e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.0225921869277954e-06])
80%|████████ | 110/137 [01:09<00:03, 6.78it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.123407855629921e-08, 0.0167236328125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.0092895030975342e-05, 2.9949471354484558e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.0092895030975342e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.7071339186713885e-07, 0.01736006699502468])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.7071339186713885e-07, 0.01736006699502468])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.7071339186713885e-07, 0.01736006699502468])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.0598450899124146e-06, 1.0598450899124146e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.0598450899124146e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.708976924419403e-07, 0.017333984375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.981536090373993e-05, 2.7237460017204285e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.981536090373993e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.3738587035259116e-07, 0.017984464764595032])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.3738587035259116e-07, 0.017984464764595032])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.3738587035259116e-07, 0.017984464764595032])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.0980293154716492e-06, 1.0980293154716492e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.0980293154716492e-06])
82%|████████▏ | 112/137 [01:10<00:03, 6.77it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.371387720108032e-07, 0.01800537109375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.8893351554870605e-05, 2.4342909455299377e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.8893351554870605e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.823425404585578e-07, 0.018503041937947273])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.823425404585578e-07, 0.018503041937947273])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.823425404585578e-07, 0.018503041937947273])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.1296942830085754e-06, 1.1296942830085754e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.1296942830085754e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.8219074010849e-07, 0.01849365234375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.9318034648895264e-05, 3.0448660254478455e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.0448660254478455e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.290323320470634e-07, 0.018892232328653336])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.290323320470634e-07, 0.018892232328653336])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.290323320470634e-07, 0.018892232328653336])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.1539086699485779e-06, 1.1539086699485779e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.1539086699485779e-06])
83%|████████▎ | 114/137 [01:10<00:03, 6.77it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.287568688392639e-07, 0.0189208984375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.9589980840682983e-05, 2.2308900952339172e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.9589980840682983e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.688165352537908e-07, 0.019209694117307663])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.688165352537908e-07, 0.019209694117307663])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.688165352537908e-07, 0.019209694117307663])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.172593329101801e-06, 1.1730007827281952e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.1730007827281952e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.691522240638733e-07, 0.01922607421875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-2.9567629098892212e-05, 2.674385905265808e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 2.9567629098892212e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.793593575413979e-07, 0.019497258588671684])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.793593575413979e-07, 0.019497258588671684])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.793593575413979e-07, 0.019497258588671684])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.1902302503585815e-06, 1.1902302503585815e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.1902302503585815e-06])
85%|████████▍ | 116/137 [01:10<00:03, 6.83it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [4.78699803352356e-07, 0.01947021484375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.034435212612152e-05, 3.03611159324646e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.03611159324646e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.852745749099995e-07, 0.019783928990364075])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.852745749099995e-07, 0.019783928990364075])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [3.852745749099995e-07, 0.019783928990364075])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2079253792762756e-06, 1.2079253792762756e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2079253792762756e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [3.855675458908081e-07, 0.019775390625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.018975257873535e-05, 2.99699604511261e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.018975257873535e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2407824669935508e-06, 0.020093949511647224])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2407824669935508e-06, 0.020093949511647224])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [1.2407824669935508e-06, 0.020093949511647224])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2265518307685852e-06, 1.226784661412239e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.226784661412239e-06])
86%|████████▌ | 118/137 [01:11<00:02, 6.79it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [1.2405216693878174e-06, 0.02008056640625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.045611083507538e-05, 3.0471011996269226e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.0471011996269226e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.278047077197698e-06, 0.020366095006465912])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.278047077197698e-06, 0.020366095006465912])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [2.278047077197698e-06, 0.020366095006465912])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2442469596862793e-06, 1.2433156371116638e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2442469596862793e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [2.2798776626586914e-06, 0.0203857421875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-3.0482187867164612e-05, 3.0471011996269226e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 3.0482187867164612e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.597550650942139e-05, 0.02051917091012001])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.597550650942139e-05, 0.02051917091012001])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.597550650942139e-05, 0.02051917091012001])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2498348951339722e-06, 1.250067725777626e-06])
87%|████████▋ | 119/137 [01:11<00:02, 6.72it/s]
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.250067725777626e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [4.595518112182617e-05, 0.0205078125])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5256926417350769e-05, 1.5253201127052307e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5256926417350769e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011528790491865948, 0.02069881744682789])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011528790491865948, 0.02069881744682789])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011528790491865948, 0.02069881744682789])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.257285475730896e-06, 1.257285475730896e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.257285475730896e-06])
88%|████████▊ | 121/137 [01:11<00:02, 6.68it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00011527538299560547, 0.02069091796875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5251338481903076e-05, 1.5251338481903076e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5251338481903076e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012256915215402842, 0.02085903473198414])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012256915215402842, 0.02085903473198414])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012256915215402842, 0.02085903473198414])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2665987014770508e-06, 1.2665987014770508e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2665987014770508e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00012254714965820312, 0.0208740234375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5255063772201538e-05, 1.5256926417350769e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5256926417350769e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012347922893241048, 0.020990710705518723])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012347922893241048, 0.020990710705518723])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012347922893241048, 0.020990710705518723])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2740492820739746e-06, 1.2740492820739746e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2740492820739746e-06])
90%|████████▉ | 123/137 [01:11<00:02, 6.75it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00012350082397460938, 0.02099609375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011574204108910635, 0.021114930510520935])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011574204108910635, 0.021114930510520935])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011574204108910635, 0.021114930510520935])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.282431185245514e-06, 1.282431185245514e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.282431185245514e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.0001157522201538086, 0.0211181640625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00010591661703074351, 0.021157030016183853])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00010591661703074351, 0.021157030016183853])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00010591661703074351, 0.021157030016183853])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2852251529693604e-06, 1.2852251529693604e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2852251529693604e-06])
91%|█████████ | 125/137 [01:12<00:01, 6.75it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00010585784912109375, 0.021148681640625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00010055536404252052, 0.021238580346107483])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00010055536404252052, 0.021238580346107483])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00010055536404252052, 0.021238580346107483])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2908130884170532e-06, 1.2908130884170532e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2908130884170532e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00010061264038085938, 0.021240234375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.5256926417350769e-05, 1.5256926417350769e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.5256926417350769e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.501005843048915e-05, 0.021339144557714462])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.501005843048915e-05, 0.021339144557714462])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.501005843048915e-05, 0.021339144557714462])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.2973323464393616e-06, 1.2973323464393616e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.2973323464393616e-06])
93%|█████████▎| 127/137 [01:12<00:01, 6.54it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [9.500980377197266e-05, 0.021331787109375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.5256926417350769e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.239320934284478e-05, 0.021493392065167427])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.239320934284478e-05, 0.021493392065167427])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.239320934284478e-05, 0.021493392065167427])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3075768947601318e-06, 1.3075768947601318e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3075768947601318e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [9.238719940185547e-05, 0.021484375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.659071656642482e-05, 0.02158947102725506])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.659071656642482e-05, 0.02158947102725506])
93%|█████████▎| 128/137 [01:12<00:01, 6.54it/s]
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.659071656642482e-05, 0.02158947102725506])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3131648302078247e-06, 1.3131648302078247e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3131648302078247e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [9.655952453613281e-05, 0.021575927734375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011202471796423197, 0.02169055864214897])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011202471796423197, 0.02169055864214897])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011202471796423197, 0.02169055864214897])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.317821443080902e-06, 1.317821443080902e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.317821443080902e-06])
95%|█████████▍| 130/137 [01:12<00:01, 6.65it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00011205673217773438, 0.021697998046875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011893160990439355, 0.021813729777932167])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011893160990439355, 0.021813729777932167])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011893160990439355, 0.021813729777932167])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3243407011032104e-06, 1.3245735317468643e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3245735317468643e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00011897087097167969, 0.021820068359375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012292816245462745, 0.021921539679169655])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012292816245462745, 0.021921539679169655])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00012292816245462745, 0.021921539679169655])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3317912817001343e-06, 1.3317912817001343e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3317912817001343e-06])
96%|█████████▋| 132/137 [01:13<00:00, 6.67it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.00012302398681640625, 0.02191162109375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011257819278398529, 0.022022686898708344])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011257819278398529, 0.022022686898708344])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [0.00011257819278398529, 0.022022686898708344])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.337612047791481e-06, 1.3383105397224426e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3383105397224426e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [0.0001125335693359375, 0.02203369140625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.687487181508914e-05, 0.02211008034646511])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.687487181508914e-05, 0.02211008034646511])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [9.687487181508914e-05, 0.02211008034646511])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.344829797744751e-06, 1.344829797744751e-06])
97%|█████████▋| 133/137 [01:13<00:00, 6.64it/s]
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.344829797744751e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [9.691715240478516e-05, 0.022125244140625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.483119017910212e-05, 0.022204697132110596])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.483119017910212e-05, 0.022204697132110596])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [8.483119017910212e-05, 0.022204697132110596])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3504177331924438e-06, 1.3506505638360977e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3506505638360977e-06])
99%|█████████▊| 135/137 [01:13<00:00, 6.54it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [8.487701416015625e-05, 0.022216796875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [7.81639973865822e-05, 0.022334547713398933])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [7.81639973865822e-05, 0.022334547713398933])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [7.81639973865822e-05, 0.022334547713398933])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3597309589385986e-06, 1.3597309589385986e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3597309589385986e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [7.82012939453125e-05, 0.0223388671875])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.5256926417350769e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.789911276428029e-05, 0.022464925423264503])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.789911276428029e-05, 0.022464925423264503])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [6.789911276428029e-05, 0.022464925423264503])
99%|█████████▉| 136/137 [01:13<00:00, 6.55it/s]
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3671815395355225e-06, 1.368112862110138e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.368112862110138e-06])
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [6.794929504394531e-05, 0.0224609375])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
q [histogram for source]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.750141306431033e-05, 0.02260897494852543])
q [histogram for baseline]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.750141306431033e-05, 0.02260897494852543])
q [histogram for decompressed: LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.510151931455766e-07, 4.082737177668605e-06], current: [4.750141306431033e-05, 0.02260897494852543])
q [histogram for metric: difference for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-2.034994395216927e-10, 2.0372681319713593e-10], current: [-1.3783574104309082e-06, 1.3783574104309082e-06])
q [histogram for metric: absolute error for LinQuantization @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 2.0372681319713593e-10], current: [0.0, 1.3783574104309082e-06])
100%|██████████| 137/137 [01:13<00:00, 1.85it/s]
q [histogram for decompressed: Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [7.511116564273834e-07, 4.082918167114258e-06], current: [4.750490188598633e-05, 0.022613525390625])
q [histogram for metric: difference for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [-1.862645149230957e-09, 1.8621904018800706e-09], current: [-1.52587890625e-05, 1.52587890625e-05])
q [histogram for metric: absolute error for Round @ 13 bits]: min/max of chunk is outside range by >1.0%, histogram may be off (initial: [0.0, 1.862645149230957e-09], current: [0.0, 1.52587890625e-05])
73.95539712905884 s
Lineplot: evaluate the effect of bits#
[5]:
suite.lineplot(fcpy.AbsoluteError, reduction="max")
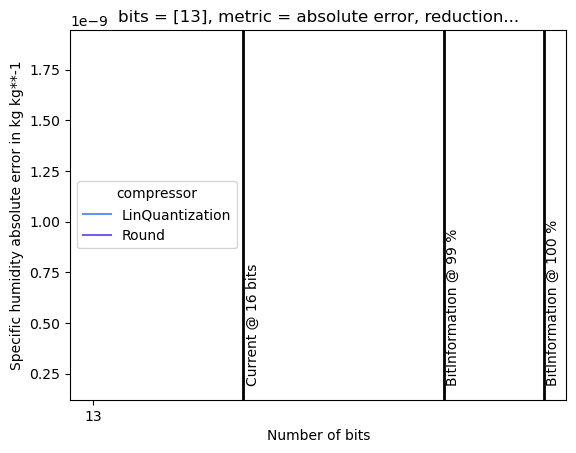
Boxplot: evaluate statistical characteristics of errors#
[6]:
bits = 13
suite.boxplot(metric=fcpy.Difference, compressor="LinQuantization", bits=bits)
suite.boxplot(metric=fcpy.Difference, compressor="Round", bits=bits)
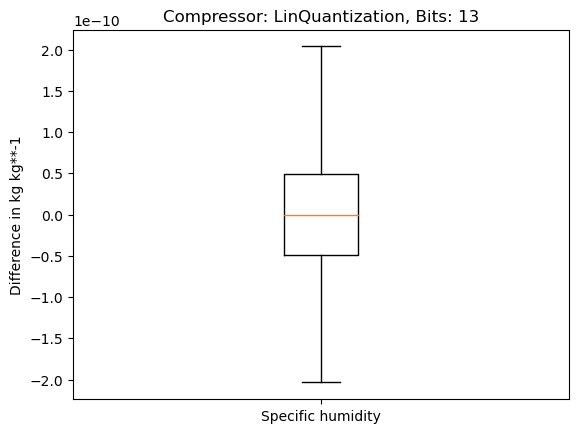
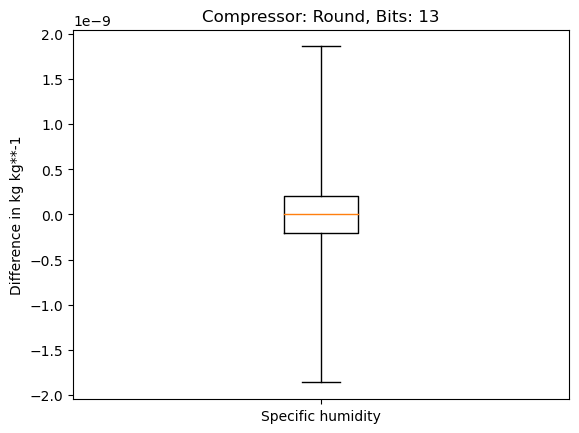
Histogram: evaluate statistical characteristics of errors#
[7]:
bits = 13
suite.histplot(metric=fcpy.Difference, compressor="LinQuantization", bits=bits)
suite.histplot(metric=fcpy.Difference, compressor="Round", bits=bits)
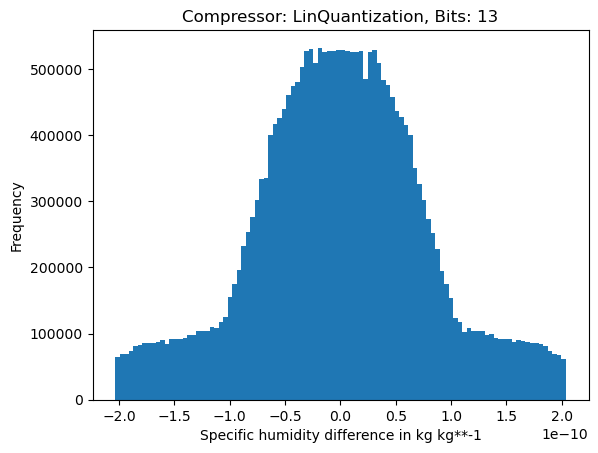
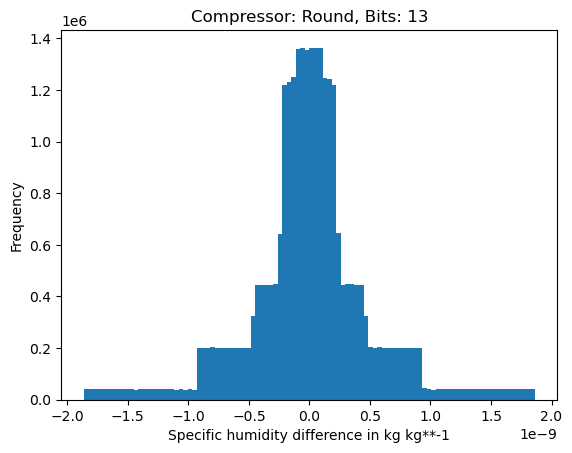
Spatial plots: evaluate the spatial distribution of errors#
[8]:
# Here we compare the spatial change of specific humidity
# by rounding values to 8 bits
fcpy.spatialplot(
ds=ds,
baseline=fcpy.Float(bits=32),
var_name="q",
compressor=fcpy.Round(bits=6),
metric=fcpy.Difference,
latitude=0,
longitude=0,
lev=1,
third_dim="lev",
)
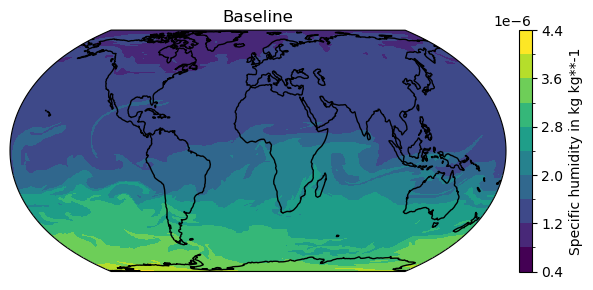
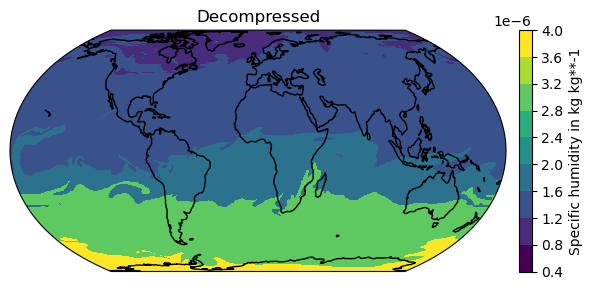
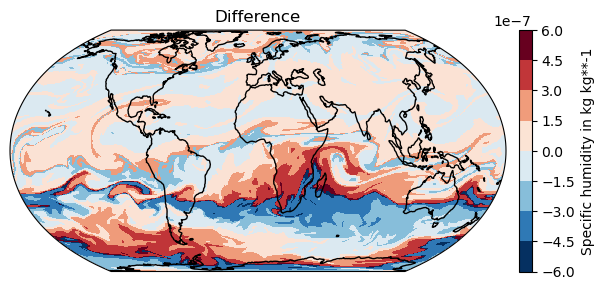

[ ]: